In this blog, we will explore “PHP Tutorial – A Comprehensive Guide to PHP Echo vs Print”. This blog covers Echo, Print, the differences between Echo and Print, along with numerous examples. Let’s dive in and check it out:
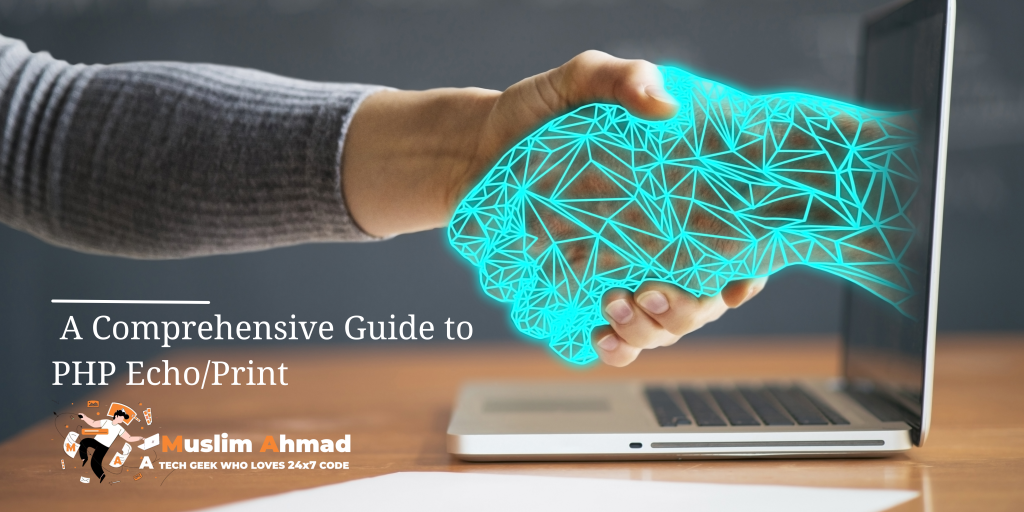
What is PHP Echo vs Print in programming languages?
In general, the terms “echo” and “print” (PHP Echo vs Print) refer to statements or functions in programming languages that are used to display or output text or data to the user or a console.
- Echo: In various programming languages, including PHP, JavaScript, and Unix shell scripting, “echo” typically refers to a command or function that outputs text or variables to the console or standard output. It is commonly used for debugging purposes or to display information to the user. The specific syntax and usage may vary between languages, but the underlying purpose remains the same.
- Print: The “print” statement or function, found in languages such as PHP, Python, and Perl, is used to display output to the user or a file. It typically takes one or more arguments, such as strings or variables, and outputs them as text. The output may be sent to the console, a file, or other output streams depending on the language and context.
Both “echo” and “print” (PHP Echo vs Print) serve similar purposes in different programming languages, allowing developers to display information, debug code, or provide feedback to users during program execution. The specific syntax and usage may vary, so it’s important to consult the documentation of the specific language you are working with for precise details.
As we know, “Echo“ and “Print“ (PHP Echo vs Print) are both keywords used to print statements in PHP Language, so now let’s check about Echo/Print in PHP Language in more detail.
What is ECHO/PRINT in PHP?
In PHP, both echo and print (PHP Echo vs Print) are used for displaying output to the browser or command line. They are language constructs rather than functions, so they can be used with or without parentheses.
- echo: The echo statement is used to output one or more strings or variables. It has a shorthand syntax and can output multiple expressions separated by commas. For example:
echo "Hello, World!"; // Output: Hello, World! $name = "John"; echo "Hello, " . $name . "!"; // Output: Hello, John!
- print: The print statement is similar to echo and is used to display output as well. It takes only one argument and returns a value of 1, which can be used in expressions. It does not support the shorthand syntax and has a slightly slower execution compared to echo. Here’s an example:
print "Hello, World!"; // Output: Hello, World! $name = "John"; print "Hello, " . $name . "!"; // Output: Hello, John!
Both echo and print (PHP Echo vs Print) are commonly used for outputting HTML content, generating dynamic web pages, and displaying variables or messages to the user. They serve a similar purpose, but echo is more widely used due to its simpler syntax and slightly better performance.
A Best Understanding about PHP Echo Vs Print
PHP Echo:
Certainly! In PHP, the echo statement is used to output strings or variables to the browser or command line. It is one of the most commonly used constructs for displaying content in PHP scripts. Here are some key details about echo in PHP:
- Syntax: The syntax of echo is simple. It can be used with or without parentheses. Example 1: Using echo without parentheses:
echo "Hello, World!";
Example 2: Using echo with parentheses:
echo("Hello, World!");
- Outputting strings: You can directly output a string within the echo statement using double quotes or single quotes. Example:
echo "Hello, World!"; // Output: Hello, World!
- Outputting variables: You can also output the value of a variable using echo. Variables can be concatenated with strings using the concatenation operator (.) or embedded within double quotes. Example 1: Using concatenation operator:
$name = "John"; echo "Hello, " . $name . "!"; // Output: Hello, John!
Example 2: Using double quotes with embedded variable:
$name = "John"; echo "Hello, $name!"; // Output: Hello, John!
- Multiple expressions: The echo statement can output multiple expressions separated by commas. It automatically converts non-string values to strings. Example:
$x = 10; $y = 5; echo "The sum of $x and $y is", $x + $y; // Output: The sum of 10 and 5 is 15
- Return value: echo does not have a return value. It directly outputs the content to the output stream.
- Performance: echo is generally faster than print because it is a language construct, whereas print is a function.
Overall, echo is a versatile and commonly used statement in PHP for outputting content. It can output both static strings and dynamically generated content using variables and expressions.
PHP Print:
Certainly! In PHP, the print statement is another way to output strings or variables to the browser or command line. It serves a similar purpose to echo but has a few differences. Here are more details about print in PHP:
- Syntax: The syntax of print is straightforward. It is used as a language construct and can be used with or without parentheses. Example 1: Using print without parentheses:
print "Hello, World!";
Example 2: Using print with parentheses:
print("Hello, World!");
- Outputting strings: Similar to echo, you can directly output a string within the print statement using double quotes or single quotes. Example:
print "Hello, World!"; // Output: Hello, World!
- Outputting variables: You can output the value of a variable using print as well. Variables can be concatenated with strings using the concatenation operator (.) or embedded within double quotes. Example 1: Using concatenation operator:
$name = "John"; print "Hello, " . $name . "!"; // Output: Hello, John!
Example 2: Using double quotes with embedded variable:
$name = "John"; print "Hello, $name!"; // Output: Hello, John!
- Single argument: Unlike echo, print accepts only a single argument. It cannot output multiple expressions separated by commas. Example:
$x = 10; $y = 5; print "The sum of $x and $y is " . ($x + $y); // Output: The sum of 10 and 5 is 15
- Return value: print has a return value of 1, which can be used in expressions. This allows you to use it within larger expressions or assign the return value to a variable. Example:
$result = print "Hello, World!"; // Output: Hello, World! echo $result; // Output: 1
- Performance: print is generally slightly slower than echo because it is a language construct that behaves like a function.
While print and echo (PHP Echo vs Print) serve similar purposes, echo is more commonly used in PHP due to its simpler syntax and slightly better performance. However, print can still be used effectively when you need to capture its return value or when working with single arguments.
Difference between Echo & Print in PHP?
In PHP, echo and print (PHP Echo vs Print) are both used to output strings or variables to the browser or command line. Here are the main differences between echo and print (PHP Echo vs Print) in PHP:
- Syntax: echo can be used with or without parentheses, while print is a language construct that always requires parentheses but also used without parentheses. Example 1: Using echo without parentheses:
echo "Hello, World!";
Example 2: Using print with parentheses:
print("Hello, World!");
- Outputting strings: Both echo and print (PHP Echo vs Print) can output a string directly. Example:
echo "Hello, World!"; // Output: Hello, World! print "Hello, World!"; // Output: Hello, World!
- Outputting variables: Both echo and print (PHP Echo vs Print) can output the value of a variable. Example:
$name = "John"; echo "Hello, $name!"; // Output: Hello, John! print "Hello, $name!"; // Output: Hello, John!
- Multiple arguments: echo can output multiple expressions separated by commas, while print can only output a single expression. Example:
$x = 10; $y = 5; echo "The sum of $x and $y is", $x + $y; // Output: The sum of 10 and 5 is 15 print "The sum of $x and $y is " . ($x + $y); // Output: The sum of 10 and 5 is 15
- Return value: echo does not have a return value, while print has a return value of 1. This means you can use print as part of an expression or assign its return value to a variable. Example:
$result = print "Hello, World!"; // Output: Hello, World! echo $result; // Output: 1
- Performance: echo is generally faster than print because it is a language construct, whereas print is a function.
Overall, echo and print (PHP Echo vs Print) are very similar in terms of functionality. The main differences are in their syntax and handling of multiple expressions. In general, echo is more commonly used in PHP due to its simpler syntax and slightly better performance, while print can be useful when you need to capture its return value or when working with single expressions.
Conclusion
In conclusion, both echo and print (PHP Echo vs Print) are language constructs in PHP that are used to output strings or variables to the browser or command line. They have similar functionalities, but there are a few differences between the two.
PHP Echo vs Print
echo is more commonly used in PHP due to its simplicity and widespread usage. It can output multiple expressions separated by commas, making it convenient for concatenating strings and variables. echo does not have a return value, but it directly outputs the content to the output stream. Since echo is a language construct, it has slightly better performance compared to print, which is a function.
print, on the other hand, accepts a single argument and always requires parentheses for multiple arguments. It has a return value of 1, which allows it to be used within larger expressions or assigned to variables. While print is less commonly used in PHP, it can be useful when you need to capture its return value or when working with single arguments.
In summary, echo and print (PHP Echo vs Print) are both useful in different scenarios, and it’s up to the developer to choose which one to use based on their specific requirements.
Check out another blog: