In this blog, we will explore “PHP Tutorial – A Comprehensive Guide to PHP Variables”. This blog covers topics such as PHP variables, PHP data types, and PHP variables call by value and reference. Let’s dive in and check it out:
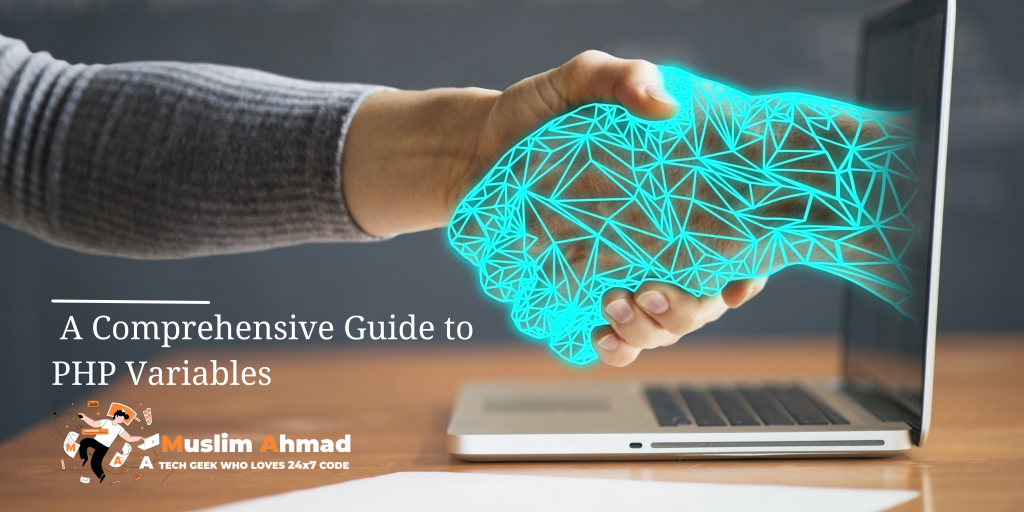
What are Variables in Programming Language?
Variables in a programming language are a named storage location in the computer’s memory that is used to hold a value. The value stored in a variable can be of various types, such as a number, a string of text, or a Boolean (true or false) value. The value stored in a variable can also be changed during the execution of a program.
When you create a variable, you must also give it a name, which is used to refer to the variable in your code. The rules for naming a variable depend on the programming language you’re using. In some languages, variable names must start with a letter, while in others they must start with a specific character (such as a dollar sign in PHP Variables).
The main benefit of using variables is that they allow you to store and manipulate data in your code. For example, you can create a variable to hold a user’s name, and then use that variable in various places in your code to display the user’s name in different ways.
When you assign a value to a variable, the value is stored in memory and the variable is said to be initialized. Once a variable is initialized, you can use it in various ways such as performing mathematical operation, using it in conditions and loops, using it to create new variables and so on.
It is a fundamental concept in programming and used in almost every programming language.
PHP Variables:
PHP variables are used to store values, such as numbers, strings, and other types of data, which can be used and manipulated in your code. Here are a few key points to keep in mind when working with PHP variables:
- PHP Variables are represented by a dollar sign ($) followed by the variable name.
- The variable name must start with a letter or an underscore, and can only contain letters, numbers, and underscores.
- PHP is a loosely typed language, which means you do not have to specify the data type of a variable when you declare it. The data type of a variable is determined by the value it is assigned.
- You can assign a value to a variable using the assignment operator (=), like this:
$name = "John Doe";
- You can also use variables in expressions and in output by concatenating with string like this
echo "My name is " . $name . " and I am " . $age . " years old";
- You can update the value of a variable at any time in your code by simply assigning a new value to it
$name = "Jane Doe";
- It’s a good practice to always initialize variables before use them, for example
$age = null;
- To check the type of variable that you have, you can use gettype() or var_dump() functions which are prebuilt functions
$age = 25; echo gettype($age); // integer
$name = "John Doe"; var_dump($name); // string(8) "John Doe"
PHP Data Types:
PHP supports several data types, which can be broadly grouped into two categories: scalar and compound types.
Scalar types:
- Integers: These are whole numbers, such as -2, 0, and 42. They can be positive or negative and are often used to represent counts or measurements.
- Floats (Doubles): These are numbers with a decimal point, such as 3.14 or -2.5. They are often used to represent measurements with a fractional component.
- Strings: These are sequences of characters, such as “Hello World!” or “123”. They are often used to represent text.
- Booleans: These are values that represent true or false. They are often used to represent the result of a comparison or test.
Compound types:
- Arrays: These are ordered collections of values, which can be of any type. They are often used to represent lists of items or data in a specific format like tables.
- Objects: These are instances of a class, which is a user-defined data type that can have properties and methods. Objects are used to represent real-world entities or complex data in an object-oriented program.
And also:
- NULL: This is a special type used to indicate that a variable has no value. It is used when you want to unset the value of a variable and can also be used to check if a variable has a value or not
Additionally, PHP also has a few other types like Resources which are a special PHP Variables that holds a reference to an external resource, like a file handle, or a database connection.
It’s worth noting that the data type of PHP Variables is determined automatically based on the value that is assigned to it. You can also explicitly cast a variable to a different data type using the casting operators like (int), (float), (string) and (bool).
Types of Variables PHP:
In PHP, there are three types of PHP Variables:
- Superglobals: These are special built-in variables that are available in all scopes throughout a script. They include PHP variables like $_GET, $_POST, $_REQUEST, $_COOKIE, $_SESSION, $_FILES, $_ENV, $_SERVER and $GLOBALS. Superglobals are automatically created by PHP and can be accessed from any function, class, or file without the need to declare them. They’re typically used to access global information such as form data and server information.
- Global variables: These are PHP variables that are defined outside of any function or class. They can be accessed from any function, class, or file within the same script. We can access user defined global variables through Superglobals variable $GLOBALS in anywhere script of PHP. To access a global variable from within a function, you must use the global keyword:
$x = 5; //global variable function test() { global $x; echo $x; } test();
- Local variables: These are PHP variables that are defined within a function or class. They are only accessible within the scope of that function or class. Once the function or class is finished executing, the variables are destroyed.
function test() { $local_var = 5; echo $local_var; } test(); echo $local_var; // this will result in an error as $local_var is not defined
When creating PHP Variables, you don’t need to explicitly specify which type of variable you’re creating. PHP will automatically determine the type based on the value that is assigned to the variable.
It’s worth noting that you should use a meaningful name for your variable and must follow the naming conventions of the language, most of the programming languages follow camel case and snake case naming conventions.
Scope of PHP Variables:
In PHP, PHP variables types refer to the data types that variables can hold, such as integers, strings, arrays, etc. Scope, on the other hand, refers to the area of the script where a variable can be accessed.
PHP Variables also have a defined scope, which determines where in the code they can be accessed. The main types of scope in PHP variables are:
- Local scope: PHP Variables defined within a function or class have local scope, which means they can only be accessed within that function or class. Once the function or class is finished executing, the variables are destroyed.
function test() { $local_var = 5; echo $local_var; } test(); echo $local_var; // this will result in an error as $local_var is not defined
- Global scope: PHP Variables defined outside of any function or class have global scope, which means they can be accessed from any function, class, or file within the same script. To access a global variable from within a function, you must use the global keyword.
$x = 5; //global variable function test() { global $x; echo $x; } test();
- Superglobal scope: Superglobal variables are special built-in variables that are available in all scopes throughout a script. Superglobals are automatically created by PHP and can be accessed from any function, class, or file without the need to declare them.
- Static scope: PHP Variables defined as static within a function retain their value even after the function has completed execution. this type of scope is created using the static keyword
function test() { static $static_var = 0; $static_var++; echo $static_var; } test(); test(); test();
It’s important to keep in mind the scope of a variable when working with it in your code, to avoid unexpected results or errors. Also you should use a meaningful variable name to indicate its scope and avoid naming conflicts.
PHP Variables Call by Value & Reference:
In PHP, function arguments can be passed in two ways: by value or by reference.
When a variable is passed by value, a copy of its value is passed to the function. Any changes made to the variable inside the function do not affect the original variable outside of the function.
$x = 5; function increment($x) { $x++; } increment($x); echo $x; // will output 5
On the other hand, when a variable is passed by reference, a reference to the original variable is passed to the function. Any changes made to the variable inside the function directly affect the original variable outside of the function. To pass a variable by reference, you need to use & before the variable name:
$x = 5; function increment(&$x) { $x++; } increment($x); echo $x; // will output 6
In general, PHP passes function arguments by value by default, but passing a variable by reference can be more efficient or make your code easier to read, specially when dealing with large data structures. However, passing by reference can also make your code harder to understand, so it’s important to use it appropriately.
Double Dollar $$ in PHP Variables:
In PHP, when you see $$ before a variable, it is indicating that the variable is a variable variable.
A variable variable is a variable that holds the name of another variable. A variable variable takes the value of a variable and treats that as the name of a variable. For example:
$name = "x"; $$name = 5; echo $x; // will output 5
In this example, the variable $name holds the string “x”, and then $$name is used to create a new variable whose name is determined by the value of $name which is “x”, and it will be assigned the value 5.
You can also use this concept with other types of variables like arrays:
$arr = 'fruits'; $$arr = ['Apple','banana','orange']; print_r($fruits); // will output Array ( [0] => Apple [1] => banana [2] => orange )
It is worth noting that using variable variables can make code harder to read and understand, and it’s generally recommended to use arrays or objects instead, when possible. Also it’s important to be careful when using variable variables, specially when using untrusted data as it might lead to injection vulnerabilities, always sanitize inputs before using them in a variable variable.
Explicit or Implicit Type Conversion in PHP Variables:
In PHP, type conversion can be either explicit or implicit.
Explicit type conversion, also known as type casting, is when you use casting operators or built-in functions to convert a variable from one data type to another. This is a way of telling the PHP engine that you want to change the type of the variable in a specific way. For example:
$x = "5"; $y = (int)$x; echo $y; // will output 5
Casting Operators: You can use the casting operators to explicitly convert a variable to a specific data type. The casting operators are:
- (int) or (integer) for integers
- (float), (double), or (real) for floating-point numbers
- (string) for strings
- (bool) or (Boolean) for booleans
Built-in Functions: PHP also provides several built-in functions for converting variables to different data types:
- intval() for integers
- floatval() for floating-point numbers
- strval() for strings
- boolval() for booleans
Example :
$str = "123"; $int = intval($str); // $int = 123 $str = "3.14"; $float = floatval($str); // $float = 3.14 $bool = boolval($str); // $bool = true
- settype() is another function that allow you to change the type of the variable to int, float, string, or bool
Example:
$var = "5"; settype($var, "integer"); settype($var, "float"); settype($var, "string"); settype($var, "Boolean"); $x = array('a' => 'apple', 'b' => 'banana'); settype($x, 'object');
Also, you can use json_encode and json_decode to convert between arrays and JSON(Javascript Object Notation) strings.
Implicit type conversion, also known as type coercion, is when the PHP engine automatically converts a variable from one data type to another based on the context in which it is used. This happens transparently and it is not controlled by the developer. For example:
$x = 5; $y = "The value of x is " . $x; echo $y; // will output "The value of x is 5"
In this case, the integer $x is implicitly converted to a string so that it can be concatenated with the other string.
Here are a few more examples of implicit type conversion in PHP:
- When performing a comparison between a string and a number, PHP will try to convert the string to a number first:
$x = "5"; $y = 5; if ($x == $y) { echo "Equal"; } else { echo "Not equal"; }
- When a string is used in a numeric context, it will be treated as a number if possible
$x = "5"; $y = $x * 2; echo $y; // will output 10 $x = "hello"; $y = $x * 2; echo $y; // will output 0
- When an array is used in a string context, it will be converted to the string “Array”
$x = ["apple", "banana", "orange"]; echo "Fruits: $x"; // will output "Fruits: Array"
- When an array is used in a numeric context, it will be treated as 0
$x = ["apple", "banana", "orange"]; $y = $x * 2; echo $y; // will output 0
It’s worth noting that implicit type conversion can lead to unexpected results, especially when dealing with strings and numbers, for example, if you want to check equality of two variables but one of them is string and the other one is number, you need to make sure that you convert them to the same data type before comparing.
It’s recommended to use explicit type conversion when necessary, to make your code more predictable and avoid hidden bugs, but also be careful when doing so because of possible data loss.
Conclusion of PHP Variables:
PHP variables are used to store and manipulate data in a script. They can be of different types like integers, floats, strings, booleans, arrays, and objects. PHP automatically determines the type of a variable based on the value assigned to it, and also, allows explicit casting to other types.
The scope of a variable defines where in the code it can be accessed, it can be Local scope, Global scope, Superglobal scope, and Static scope. And, by default, variables are passed by value, but also, PHP allows passing a variable by reference, by prefixing the & before the variable name.
Additionally, PHP has a feature called variable variables, which allows creating a variable whose name is determined by the value of another variable, this feature is indicated by using two $ before the variable name. It’s important to note that the use of variable variables can make the code hard to read and understand and it’s recommended to use arrays or objects instead, if possible.
Overall, PHP Variables are a fundamental concept in programming and PHP provides a rich set of features to work with them effectively, and understanding how to properly use them can help you write more efficient and readable code.
Check out my Another Blog: