In this blog, we will explore “PHP Tutorial – A Comprehensive Guide to PHP Introduction”. This tutorial covers all key topics, including PHP Introduction, PHP Common Uses, History of PHP Language, and more. Let’s dive in and check it out:
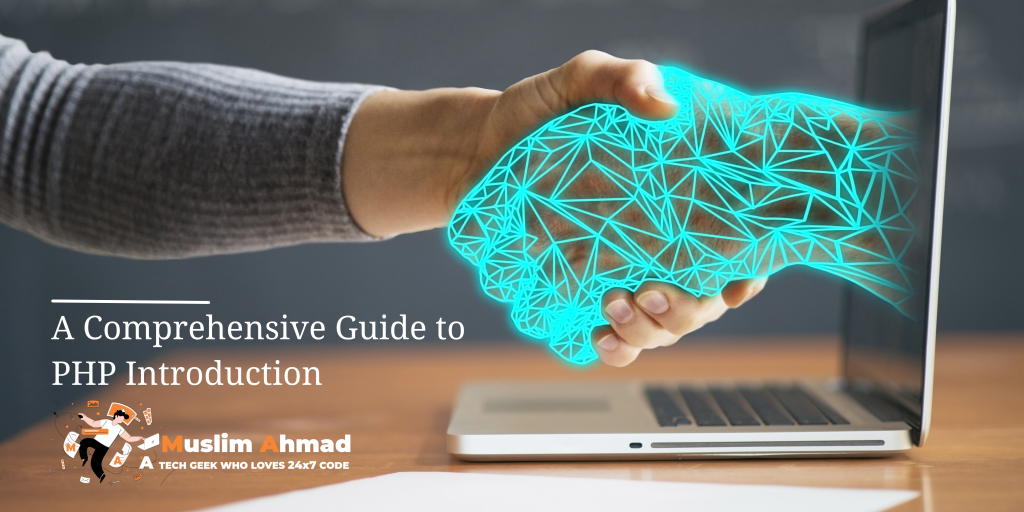
PHP Introduction:
PHP is a popular server-side scripting language that is widely used for web development. It is particularly well-suited for creating dynamic and interactive websites.
PHP code is executed on the server, rather than in the user’s web browser, which makes it a good choice for tasks such as processing form data, storing and retrieving data from a database, and generating dynamic content.
PHP is an open-source language, which means that it is freely available for anyone to use and modify. It is also easy to learn, especially for those with a background in programming, and there is a large and active community of developers who contribute to the language and create tools and resources that can be used with PHP.
PHP is a general-purpose programming language, which means that it can be used to create a wide range of applications, from simple websites to complex web-based systems. It is particularly well-suited for creating websites that need to store and retrieve data from a database, or that need to interact with users in real-time.
PHP is often used in combination with other technologies, such as MySQL (a database management system) and HTML (a markup language for creating web pages). It can also be used to integrate with other systems and platforms, such as payment processors, social media APIs, and more.
PHP is used to create the backend of a web application and can be integrated with HTML, CSS, and JavaScript to create a complete web application. It is often used in conjunction with a database management system, such as MySQL, to store and retrieve data for a web application
Some of the key features of PHP include:
- It is open-source and freely available.
- It is easy to learn, especially for those with a background in programming.
- It has a large and active community, with a wealth of libraries, frameworks, and resources available.
- It is highly flexible and can be used to create a wide range of applications, from simple websites to complex web-based systems.
Here is a simple “Hello, World!” script in PHP:
To run this script, you will need to have a web server with PHP installed. You can then place the script in a file with a .php extension, and access it through your web browser.
<?php echo "Hello, World!"; ?>
For example, if you save the script in a file called “hello.php”, you can access it by entering the following URL in your web browser:
http://localhost/hello.php
This will display the message “Hello, World!” in your web browser.
Here is a breakdown of what is happening in the script:
- The <?php and ?> tags indicate the start and end of a PHP block. Everything between these tags is interpreted as PHP code.
- The echo statement is used to output a string to the web browser. In this case, it is outputting the string “Hello, World!”.
- The semicolon (;) at the end of the line indicates the end of the PHP statement.
This is a very basic PHP script, but it illustrates some of the basic concepts of the language, such as how to output text to the web browser and how to use PHP tags to enclose PHP code.
Here are some basic concepts and techniques that you can use to get started with PHP:
- PHP Syntax: This refers to the rules and conventions that you need to follow when writing PHP code. For example, PHP statements must be terminated with a semicolon, and code blocks are enclosed in curly braces.
- Variables: A variable is a named container that you can use to store data in your PHP scripts. You can use variables to store numbers, strings, arrays, and other data types.
- Operators: Operators allow you to perform basic operations such as assignment, arithmetic, and comparison. For example, you can use the “+” operator to add two numbers together, or the “==” operator to compare two values for equality.
- Control Structures: Control structures allow you to control the flow of execution in your PHP scripts. For example, you can use an “if” statement to execute a block of code only if a certain condition is met, or a “for” loop to repeat a block of code a specified number of times.
- Functions: A function is a reusable piece of code that you can call from anywhere in your PHP scripts. You can use functions to perform a specific task, or to return a value to the calling code.
- Arrays: An array is a data structure that allows you to store a collection of values under a single name. You can use arrays to store lists of data, such as a list of names or a list of prices.
- Strings: A string is a sequence of characters, such as a word or a sentence. You can use strings to store and manipulate text data in your PHP scripts.
- Forms and User Input: You can use PHP to process data that is submitted through an HTML form on a web page. This can be used to create interactive websites that allow users to enter and submit data.
There are many more advanced concepts and techniques in PHP, but these are some of the basics that you can use to get started. You can learn from next tutorials.
Here is a brief tutorial on some of the basic features of PHP:
- Variables: In PHP, variables are denoted with a dollar sign ($). For example:
$name = "John"; $age = 30;
- Data types: PHP supports a variety of data types, including integers, floating-point numbers, strings, and Booleans. You can use the var_dump function to output the data type and value of a variable:
$name = "John"; var_dump($name); // string(4) "John" $age = 30; var_dump($age); // int(30) $is_admin = true; var_dump($is_admin); // bool(true)
- Arrays: PHP supports both indexed and associative arrays. Here is an example of an indexed array:
$fruits = array("apple", "banana", "cherry"); echo $fruits[0]; // apple
And here is an example of an associative array:
$person = array("name" => "John", "age" => 30, "is_admin" => true); echo $person["name"]; // John
- Control structures: PHP supports various control structures, such as if statements, for loops, and while & do while loops. Here is an example of an if statement:
$age = 30; if ($age > 21) { echo "You are allowed to enter the venue."; } else { echo "You are not allowed to enter the venue."; }
And here is an example of a for loop:
for ($i = 0; $i < 10; $i++) { echo $i; }
- Functions: You can define your own functions in PHP. Here is an example of a function that calculates the area of a rectangle:
$age = 30; function calculateArea($width, $height) { return $width * $height; } $area = calculateArea(10, 20); echo $area; // 200
This is just a brief introduction to some of the basic features of PHP. There is much more to learn, including working with forms, handling user input, connecting to databases, and creating dynamic web pages.
PHP’s Common Uses:
PHP is a versatile and widely-used language that is well-suited for a wide range of applications. Some common uses of PHP include:
- Web development: PHP is often used to build dynamic and interactive websites. It can be used to create web pages that are customized based on user input, or that display data from a database.
- E-commerce: PHP is a popular choice for building online stores and other e-commerce platforms. It can be used to handle transactions, manage inventory, and process orders.
- Content management systems: PHP is often used to build content management systems (CMS) that allow users to easily create, edit, and publish content on a website.
- Server-side scripting: PHP can be used to perform tasks on the server side, such as validating form data, sending emails, or generating reports.
- Integration with other systems: PHP can be used to interact with other systems and platforms, such as payment processors, social media APIs, and more.
- Command-line scripting: PHP can also be used to create command-line scripts that can be run from the terminal. This can be useful for automating tasks or creating utilities.
Overall, PHP is a powerful and flexible language that is well-suited for a wide range of web development projects.
History of PHP Language:
PHP (originally an acronym for “Personal Home Page“, now recursively stands for “PHP: Hypertext Preprocessor“) is a server-side scripting language that is widely used for web development. It was created by Rasmus Lerdorf in 1995, and has evolved over the years to become one of the most popular web programming languages in use today.
In the early days, PHP was primarily used to create simple scripts for personal websites, but as the language grew in popularity, it was used to build more complex web applications. In 1997, two developers, Andi Gutmans and Zeev Suraski, rewrote the language’s core, creating PHP 3. This new version of PHP included a number of improvements and new features, such as improved performance and support for object-oriented programming.
PHP 4 was released in 2000, introducing more improvements to performance and the addition of support for the HTTP sessions. PHP 4 was widely used and supported until 2008.
In 2004, PHP 5 was released, which added support for a number of new features, including support for Object-Oriented Programming (OOP) features, such as classes and objects, support for XML and improved support for databases. This version of PHP became widely used for building web applications, many are still using in the modern era.
In 2014, PHP 7 was released, introducing major changes to the language and significantly improving performance. This version of PHP is faster than previous versions, which is one of the main reasons for its adoption.
Currently, PHP 8.0 was released in Nov 2020. This version of PHP introduced a number of new features, including named arguments, match expressions, union types, attributes, and more.
PHP continues to be widely used to build web applications of all sizes, from small personal blogs to large-scale enterprise applications. The language is also well-suited for building web services and APIs. Many popular web frameworks and content management systems, such as WordPress, Drupal, and Laravel, are written in PHP. Today, PHP is widely used for web development, powering some of the world’s most popular websites, including Facebook, Wikipedia, and more.
PHP Zend Engine:
The Zend Engine is the open-source virtual machine that PHP uses to execute scripts. It was first introduced in PHP version 4, released in 2000, and has since been included in all subsequent versions of the language. The Zend Engine is responsible for memory management, language parsing, and bytecode execution.
The Zend Engine is named after the company, Zend Technologies, which was founded by Andi Gutmans and Zeev Suraski, who were the developers responsible for creating PHP 3 and rewriting the language’s core. The company continues to play an important role in the development of PHP, and the Zend Engine remains the backbone of the language.
One of the key features of the Zend Engine is its just-in-time (JIT) compilation capabilities, which allows it to dynamically translate PHP scripts into machine code at runtime. This improves performance, as it eliminates the need for the interpreter to parse and execute the same code multiple times.
The Zend Engine also includes a number of other performance-enhancing features, such as a memory manager and opcode caching. The memory manager is responsible for managing memory used by PHP scripts and ensuring that it is allocated and deallocated efficiently. The opcode caching, allows to store precompiled scripts in memory, so that they don’t have to be parsed and compiled each time they are executed. This reduces the time it takes for scripts to run and helps to improve performance.
Overall, the Zend Engine plays a critical role in the performance and functionality of PHP, and its inclusion in the language has helped to make PHP one of the most popular web programming languages in use today.
Pros & Cons
PHP also has some pros and cons that you should consider when deciding whether to use it for your project.
Pros of using PHP:
- Open-source and widely available: PHP is an open-source language that is freely available to use and distribute. This makes it a cost-effective choice for developers.
- Large and active community: PHP has a large and active community of developers, which means that there is a wealth of resources, libraries, frameworks, and support available.
- Easy to learn: PHP is relatively easy to learn, especially for those with a background in programming. It has a simple syntax and there are many resources available for learning the language.
- Highly flexible: PHP can be used to create a wide range of applications, from simple websites to complex web-based systems. It is highly flexible and can be integrated with other technologies and systems.
Cons of using PHP:
- Performance: PHP can be slower than some other languages, such as C or C++, especially for applications that require a lot of CPU or memory resources.
- Security vulnerabilities: Like any language, PHP has the potential for security vulnerabilities if it is not used properly. It is important to follow best practices and keep your PHP code up-to-date to minimize the risk of security issues.
- Lack of strong typing: PHP is a dynamically-typed language, which means that variables do not have a fixed type. This can make it harder to catch type-related errors at compile-time, and can lead to issues if the wrong type of data is passed to a function or method.
Overall, PHP has many pros and is a popular choice for web development projects, but it is important to carefully consider its potential cons and how they might affect your specific project.
PHP Frameworks & CMS
PHP frameworks and content management systems (CMS) are tools that provide a set of libraries and tools to help developers build web applications more quickly and efficiently. Some popular PHP frameworks and CMS include:
- PHP frameworks:
- Laravel: Laravel is a popular PHP framework that is designed to be expressive, elegant, and easy to use. It offers a wide range of features, including a routing system, a template engine, an ORM (object-relational mapper), and a command-line interface (CLI).
- Yii Framework: Yii is a high-performance PHP framework that is designed to be fast, secure, and flexible. It is especially well-suited for developing large-scale web applications, such as portals, forums, content management systems, and e-commerce platforms.
- CodeIgniter: CodeIgniter is a lightweight PHP framework that is known for its simple installation and configuration process. It offers a range of libraries and helpers to make it easier to build web applications, including support for forms, sessions, and database management.
- Symfony: Symfony is a full-stack PHP framework that offers a wide range of components and tools to help developers build web applications more quickly and efficiently. It is used by many large-scale projects, including the Drupal CMS.
- CMS:
- WordPress: WordPress is a popular open-source CMS that is used to build websites and blogs. It is known for its ease of use, wide range of plugins and themes, and support for various types of content.
- Joomla: Joomla is another popular open-source CMS that is used to build websites, online stores, and forums. It offers a range of features, including support for multiple languages, templates, and extensions.
- Drupal: Drupal is a powerful open-source CMS that is used to build websites, intranets, and online communities. It offers a range of features, including support for multiple languages, templates, and extensions.
There are many other PHP frameworks and CMS available, each with its own set of features and target audience. You can find more detailed information and comparison of these and other PHP frameworks and CMS on the other tutorials.
What’s new in PHP 7 & PHP 8
PHP 7 and PHP 8 are major versions of PHP that introduced several new features and improvements. Here are some of the highlights:
PHP 7:
- Improved performance: PHP 7 introduced a new Zend Engine 3, which significantly improved the performance of PHP scripts.
- Error handling: PHP 7 introduced a new error handling mechanism called “Error Exception Hierarchy,” which allows developers to catch and handle different types of errors more effectively.
- New operators: PHP 7 introduced several new operators, such as the null coalescing operator (??) and the spaceship operator (<=>).
PHP 8:
- Just-in-time (JIT) compilation: PHP 8 introduced a JIT compiler that can significantly improve the performance of certain types of code.
- Union types: PHP 8 introduced the ability to specify that a variable can be of multiple types using the | operator.
- Named arguments: PHP 8 introduced the ability to specify arguments by name when calling a function, making the code more readable and easier to maintain.
- Match expressions: PHP 8 introduced a new match expression that allows developers to easily perform a series of comparisons and return a value based on the first match.
There are many other new features and improvements in both PHP 7 and PHP 8. You can find more detailed information in the official documentation & you can learn from other tutorials in more details:
- PHP 7: https://www.php.net/manual/en/migration70.new-features.php
- PHP 8: https://www.php.net/manual/en/migration80.new-features.php
Conclusion:
PHP has a large and active community of developers and users, which helps to ensure that it is well-supported and constantly evolving. As such, PHP is a good choice for web development, and is used by many large and successful websites and applications.
Check out another blog: