In this blog, we will explore “PHP Tutorial – A Comprehensive Guide to PHP Constant”. This is the place where you can learn about all aspects of PHP constants, including constant arrays, constants’ global scope, constants with the $GLOBALS superglobal array, the constant() function, the defined() function, and more. Let’s dive in and check it out:
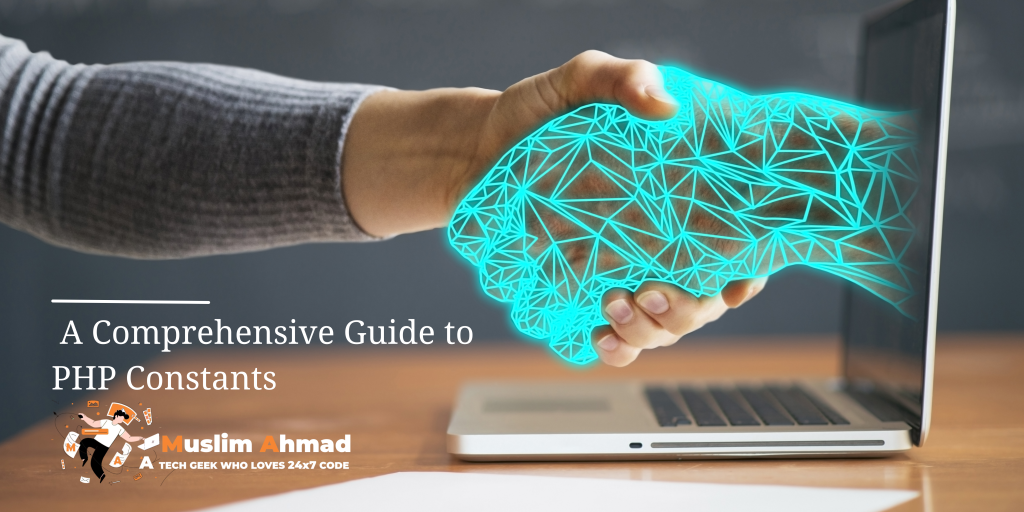
What are Constants in programming language?
In programming, the term “constant” refers to a value that remains unchanged during the execution of a program. It is a fixed value that cannot be modified once it has been assigned. Constants are used to represent data that is not intended to be modified, such as mathematical values, physical constants, or configuration settings.
Constants are typically declared and defined at the beginning of a program or within a specific scope, depending on the programming language. They are given a name or identifier and associated with a specific value. Once a constant is defined, any attempt to modify its value will result in an error or a compilation failure.
The use of constants can provide several benefits in programming, including improved code readability and maintainability. By assigning meaningful names to constants, it becomes easier to understand the purpose and significance of the associated values. Additionally, using constants allows programmers to easily change the value at a single location if needed, without having to modify multiple occurrences throughout the codebase.
Here’s an example of declaring and using constants in different programming languages:
In Python:
# Defining a constant PI = 3.14159 # Using the constant radius = 5 circumference = 2 * PI * radius print(circumference) # Output: 31.4159
In Java:
public class ConstantsExample { // Defining a constant public static final double PI = 3.14159; public static void main(String[] args) { // Using the constant int radius = 5; double circumference = 2 * PI * radius; System.out.println(circumference); // Output: 31.4159 } }
In C++:
#include <iostream> int main() { // Defining a constant const double PI = 3.14159; // Using the constant int radius = 5; double circumference = 2 * PI * radius; std::cout << circumference << std::endl; // Output: 31.4159 return 0; }
Note that the specific syntax for declaring constants may vary between programming languages, but the concept of a constant as an unmodifiable value remains consistent.
PHP Contants – A Best Understanding about PHP Constant
In PHP, PHP Constants are similar to variables, but their values cannot be changed once they are defined. Constants are often used to store values that remain constant throughout the execution of a PHP script.
Here’s the syntax for defining constants in PHP:
define(name, value, case_insensitive);
- name: Specifies the name of the constant.
- value: Specifies the value assigned to the constant.
- case_insensitive (optional): Specifies whether the constant name should be case-insensitive. By default, it is set to false.
Here’s an example that demonstrates the usage of constants in PHP:
// Defining a constant define("PI", 3.14159); // Using the constant $radius = 5; $circumference = 2 * PI * $radius; echo $circumference; // Output: 31.4159
In this example, the constant PI is defined with a value of 3.14159. The constant is then used in a calculation to find the circumference of a circle given the radius.
Some important points to note about PHP constants:
- Constant names are typically written in uppercase letters to distinguish them from variables.
- Constants are accessed without using the dollar sign ($).
- Constants can be used anywhere in a PHP script, regardless of scope.
- Constants are automatically global and can be accessed from any function or class within the script.
- Once a constant is defined, it cannot be redefined or undefined.
- Constants are case-sensitive by default, but you can make them case-insensitive by setting the third argument of the define() function to true.
Overall, PHP constants provide a way to store values that remain fixed throughout the execution of a script, offering clarity and consistency in code.
Here are a few examples to illustrate how to define constants in PHP:
define("SITE_NAME", "My Website"); define("MAX_ATTEMPTS", 3); define("PI", 3.14159, true); // Case-insensitive constant
In the first example, we define a constant named SITE_NAME with the value “My Website”. In the second example, MAX_ATTEMPTS is defined with the value 3. Finally, in the third example, we define PI with the value 3.14159 and set it as case-insensitive.
Once defined, constants can be used throughout your PHP script by simply referencing their name. For example:
echo SITE_NAME; // Output: My Website echo MAX_ATTEMPTS; // Output: 3 echo pi; // Output: 3.14159 (case-insensitive)
Note that constants do not require a dollar sign ($) prefix like variables do. You can access constants directly by their name.
It’s important to keep in mind that constants are global in scope and can be accessed from anywhere in your PHP script. However, it’s considered good practice to define constants within the appropriate scope to limit their visibility and prevent naming conflicts.
Defining constants in PHP provides you with a convenient way to store and utilize fixed values throughout your codebase, improving readability and maintainability.
Best Practices for Using PHP Constant
To make the most of PHP constants, consider the following best practices:
- Meaningful Names: Use descriptive names for constants to provide clarity and understanding. Avoid generic names or abbreviations that may lead to confusion.
- Uppercase Convention: Follow the convention of using uppercase letters for constant names to distinguish them from variables and make them stand out in the code.
- Scope Considerations: Constants are automatically global and can be accessed from any part of your PHP script. However, it’s good practice to define constants within the appropriate scope to limit their visibility and prevent potential naming conflicts.
- Documentation: Document your constants by providing comments that describe their purpose, expected values, and any relevant usage instructions. This helps other developers understand and utilize them correctly.
Benefits of Using PHP Constant
Using constants in your PHP code offers several advantages:
- Readability and Maintainability: Constants provide self-documenting code by giving meaningful names to fixed values. This enhances code readability and makes it easier to understand the purpose of specific values.
- Avoiding Magic Numbers: Constants help eliminate “magic numbers” in code. Magic numbers are hard-coded numeric values that lack context and make code maintenance challenging. By assigning them to constants, you can give them descriptive names that provide clarity and eliminate ambiguity.
- Consistency and Centralized Value Management: Constants allow you to store values in a centralized location. This makes it easy to change a value at a single point, ensuring consistency throughout your codebase.
- Protection against Accidental Modification: Once a constant is defined, it cannot be redefined or undefined within the script. This protects against accidental modification, ensuring that the value remains constant throughout the execution.
PHP Constant Arrays
In PHP, PHP Constant can also be defined as arrays, allowing you to store a collection of values that remain constant throughout your script execution. PHP Constant arrays provide a way to group related data together and access it easily using meaningful names.
To define a constant array in PHP, you can use the define() function along with the array() construct. Here’s the syntax:
define('CONSTANT_NAME', array( value1, value2, // ... ));
Let’s see an example of defining a constant array:
define('FRUITS', array( 'apple', 'banana', 'orange' ));
In this example, we define a constant array named FRUITS that contains three elements: ‘apple’, ‘banana’, and ‘orange’. Once defined, the values within the constant array cannot be modified.
You can access the elements of a constant array using their index. For example:
echo FRUITS[0]; // Output: apple echo FRUITS[1]; // Output: banana echo FRUITS[2]; // Output: orange
PHP Constant arrays can also be multidimensional, allowing you to store more complex data structures. Here’s an example:
define('MATRIX', array( array(1, 2, 3), array(4, 5, 6), array(7, 8, 9) ));
In this case, we define a PHP Constant array named MATRIX that represents a 3×3 matrix. You can access its elements using two indices:
echo MATRIX[0][0]; // Output: 1 echo MATRIX[1][2]; // Output: 6 echo MATRIX[2][1]; // Output: 8
It’s worth noting that constant arrays are immutable, meaning you cannot modify their elements or reassign a different array to the constant name.
Using constant arrays in PHP can be beneficial when you have a collection of values that should remain constant and need to be easily accessible throughout your script. They provide a structured way to organize data and enhance code readability.
Note: PHP 7 introduced PHP Constant Arrays so we cannot use it in the previous version of PHP.
Moe About PHP Constant Array:
PHP does not support the direct declaration of constant arrays using the define() function.
However, you can achieve a similar effect by defining an array as a constant-like structure using the const keyword within a class or using the define() function in combination with the serialize() and unserialize() functions. Let me explain both approaches:
- Defining an Array as a PHP Constant – like Structure within a Class:
class Constants { const FRUITS = ['apple', 'banana', 'orange']; } // Accessing the constant array echo Constants::FRUITS[0]; // Output: apple echo Constants::FRUITS[1]; // Output: banana echo Constants::FRUITS[2]; // Output: orange
By encapsulating the PHP Constant Array within a class as a constant, you can access its elements using the :: scope resolution operator.
- Defining a PHP Constant Array using define(), serialize(), and unserialize():
define('FRUITS', serialize(['apple', 'banana', 'orange'])); // Accessing the constant array $fruits = unserialize(FRUITS); echo $fruits[0]; // Output: apple echo $fruits[1]; // Output: banana echo $fruits[2]; // Output: orange
In this approach, the array is serialized using the serialize() function before assigning it to the constant. To use the constant array, you need to unserialize it using the unserialize() function.
Please note that these approaches simulate constant arrays, but they are not true constant arrays as PHP does not provide direct support for them. It’s essential to be aware of potential limitations and consider alternative solutions if your requirements demand truly immutable arrays.
Constants Global Scope
In PHP, PHP Constants are global by default, meaning they can be accessed from anywhere in your code, including within functions, classes, and even included files. Once a constant is defined, its value remains the same throughout the entire script execution, making it a useful tool for storing data that should not be modified.
To define a global PHP Constant in PHP, you can use the define() function, which takes two arguments: the name of the constant and its value. Here’s an example:
define('APP_NAME', 'My Application');
In this example, we define a global PHP constant named APP_NAME with a value of ‘My Application’. Once defined, you can access this constant from any part of your script using its name:
echo APP_NAME; // Output: My Application
This includes within functions and classes:
function displayAppName() { echo APP_NAME; } class MyClass { public function getAppName() { return APP_NAME; } } displayAppName(); // Output: My Application $obj = new MyClass(); echo $obj->getAppName(); // Output: My Application
Since constants are global by default, you can also use them across multiple files by including the file that defines the constant. For example, let’s say we have a file named constants.php that defines some constants:
define('APP_NAME', 'My Application'); define('APP_VERSION', '1.0');
We can then include this file in another PHP script:
include 'constants.php'; echo APP_NAME; // Output: My Application echo APP_VERSION; // Output: 1.0
In this example, the constants defined in constants.php are accessible from the main script after including the file.
To summarize, PHP Constants are global by default and can be accessed from anywhere in your script, including within functions, classes, and included files. This makes them a convenient way to store values that should remain constant throughout the script execution.
is it possible to access PHP Constant with $GLOBALS superglobal array?
No, PHP Constants cannot be accessed using the $GLOBALS superglobal array. The $GLOBALS array is used to access global variables in PHP, not constants.
PHP Constants are accessed directly by their name, without using the $ prefix. They are automatically accessible within the global scope and can be used anywhere in your PHP script without needing to use the $GLOBALS array.
Here’s an example to illustrate the difference:
define('SITE_NAME', 'My Website'); $globalVariable = 'Hello, World!'; function displayConstantsAndGlobalVariable() { echo SITE_NAME; // Output: My Website echo $GLOBALS['globalVariable']; // Output: Hello, World! } displayConstantsAndGlobalVariable();
In this example, the constant SITE_NAME and the global variable $globalVariable are defined. The displayConstantsAndGlobalVariable() function is then called, which echoes both the PHP constant and the global variable.
As you can see, the constant SITE_NAME is accessed directly by its name (SITE_NAME), while the global variable $globalVariable is accessed using the $GLOBALS array.
Remember that PHP constants and global variables are distinct entities in PHP, and they have different ways of being accessed.
Constant() function in PHP
In PHP, the constant() function is used to retrieve the value of a PHP constant by its name. It allows you to access the value of a PHP constant dynamically using a string representation of the constant name.
The syntax of the constant() function is as follows:
constant(name);
Here’s an example to demonstrate the usage of the constant() function:
define('SITE_NAME', 'My Website'); define('MAX_ATTEMPTS', 3); $constantName = 'SITE_NAME'; echo constant($constantName); // Output: My Website $constantName = 'MAX_ATTEMPTS'; echo constant($constantName); // Output: 3
In this example, we define two PHP constants, SITE_NAME and MAX_ATTEMPTS, with their respective values. We then use the constant() function to dynamically access their values using a string representation of their names.
The constant() function takes the constant name as an argument and returns its corresponding value. It can be helpful when you have the constant name stored in a variable or when you need to access constants dynamically based on runtime conditions.
It’s important to note that the constant() function can only retrieve the value of constants and does not work with regular variables. If you try to use it with a variable, it will throw an error.
$variableName = 'someVariable'; echo constant($variableName); // Error: constant(): Couldn't find constant 'someVariable'
In summary, the constant() function in PHP allows you to access the value of a constant by providing its name as a string argument. It provides a way to retrieve constant values dynamically, contributing to more flexible and dynamic code.
Difference between direct access & constant() function:
In PHP, there are two different ways to access the value of a constant: direct access and using the constant() function. Let’s explore the differences between these two approaches:
- Direct Access:
define('SITE_NAME', 'My Website'); echo SITE_NAME; // Output: My Website
When you directly access a constant using its name, you simply reference the constant without any additional function or syntax. This method is straightforward and efficient, allowing you to access the constant value directly. It is the most common and recommended way to access PHP constants.
- Using the constant() function:
define('SITE_NAME', 'My Website'); $constantName = 'SITE_NAME'; echo constant($constantName); // Output: My Website
The constant() function allows you to access the value of a constant by providing its name as a string argument. This method is useful when you have the constant name stored in a variable or when you need to access constants dynamically based on runtime conditions.
Unlike direct access, the constant() function allows you to evaluate the constant name dynamically at runtime. It can be helpful in situations where you need to programmatically determine the constant to access.
It’s important to note that using the constant() function introduces a slight performance overhead compared to direct access, as it involves a function call and additional processing.
In general, direct access is the preferred and most commonly used approach to access constants in PHP. It provides a more concise and efficient way to retrieve the constant value. However, the constant() function offers flexibility when you need to access constants dynamically or when the constant name is stored in a variable.
Choose the method that best suits your specific requirements and coding context
What does defined() function do for PHP Constant?
In PHP, the defined() function is used to check whether a PHP constant has been defined or not. It allows you to determine if a PHP constant with a specific name has been defined using the define() function.
The defined() function takes the constant name as a string argument and returns true if the constant is defined, and false otherwise.
Here’s an example to illustrate the usage of the defined() function:
define('SITE_NAME', 'My Website'); if (defined('SITE_NAME')) { echo 'SITE_NAME is defined'; } else { echo 'SITE_NAME is not defined'; }
In this example, we define a PHP constant named SITE_NAME with the value ‘My Website’. We then use the defined() function to check if the constant SITE_NAME is defined. Since it is defined in this case, the output will be ‘SITE_NAME is defined’.
If you attempt to use the defined() function with an undefined constant, it will return false. For example:
if (defined('UNKNOWN_CONSTANT')) { echo 'UNKNOWN_CONSTANT is defined'; } else { echo 'UNKNOWN_CONSTANT is not defined'; }
In this case, since the constant UNKNOWN_CONSTANT is not defined, the output will be ‘UNKNOWN_CONSTANT is not defined’.
The defined() function can be useful when you need to ensure that a constant is defined before using it, especially if the constant’s existence is not guaranteed. It helps prevent errors that may occur when attempting to use undefined constants.
It’s important to note that the defined() function only works with constants defined using the define() function. It does not work with built-in constants or variables.
How would be print all defined PHP Constants?
To print all the defined PHP Constants, you can use the get_defined_constants() function. This function returns an associative array that contains all the currently defined constants in PHP, including both user-defined and built-in constants.
Here’s an example that demonstrates how to print all the defined constants in PHP:
$constants = get_defined_constants(true)['user']; foreach ($constants as $constantName => $constantValue) { echo $constantName . ' => ' . $constantValue . '<br>'; }
In this example, we use the get_defined_constants(true) function to retrieve all the defined constants, including user-defined constants. We access the ‘user’ key in the returned associative array to access only the user-defined constants.
We then iterate over the $constants array using a foreach loop. For each constant, we print its name ($constantName) and its value ($constantValue) using the echo statement. In this example, we separate the constant name and value with => and use <br> to display each constant on a new line in the output.
By executing this code, you will see a list of all the user-defined constants and their corresponding values.
Note that this code will only display user-defined constants, excluding built-in constants defined by PHP or its extensions. If you also want to include built-in constants in the output, you can remove the ‘user’ key from the get_defined_constants(true) function.
$constants = get_defined_constants(true); foreach ($constants as $category) { foreach ($category as $constantName => $constantValue) { echo $constantName . ' => ' . $constantValue . '<br>'; } }
With this modification, all defined constants, including user-defined and built-in constants, will be printed.
Remember to use caution when printing constants in a production environment, as some constants may contain sensitive information.
is it possible to destroy or unset specific constant or all constants in PHP?
In PHP, it is not possible to unset or destroy a constant that has been defined using the define() function. Constants are intended to be immutable and remain constant throughout the execution of the script.
If you attempt to unset a constant using the unset() function or the unset statement, you will receive a warning and the constant will continue to exist with its original value. For example:
define('FOO', 'bar'); unset(FOO); echo FOO; // Output: 'bar'
In this example, we attempt to unset the FOO constant, but it will still be defined with its original value of ‘bar’. Attempting to use the constant after attempting to unset it will not produce an error, but it will still output the original value.
If you need to redefine a constant with a different value, you can use the define() function again to redefine the constant. This will override the previous definition of the constant with the new value.
define('FOO', 'bar'); define('FOO', 'baz'); echo FOO; // Output: 'baz'
In this example, we redefine the FOO constant with a new value of ‘baz’. The second call to define() overrides the previous definition of FOO, and the output will be ‘baz’.
In summary, constants in PHP are intended to be immutable and remain constant throughout the execution of the script. You cannot unset or destroy a constant that has been defined using the define() function, but you can redefine a constant with a new value using the same function.
Conclusion
In conclusion, constants in PHP are named values that remain constant throughout the execution of a script. They are useful for storing fixed data values that do not change during runtime, such as configuration settings, database credentials, or API keys.
Here are some of the commonly used predefined functions for constants in PHP:
- defined(): This function is used to check if a constant has been defined. It returns true if the constant is defined and false otherwise. Example: defined(‘CONSTANT_NAME’)
- constant(): This function is used to retrieve the value of a constant by its name. It allows you to access the value of a constant dynamically using a string representation of the constant name. Example: constant(‘CONSTANT_NAME’)
- get_defined_constants(): This function returns an associative array containing all the currently defined constants, including both user-defined and built-in constants. Example: get_defined_constants()
- defined() and constant() are often used together to conditionally check if a constant is defined and retrieve its value dynamically. Example:
Constants in PHP are defined using the define() function, which takes two arguments: the name of the constant and its value. Constants can be accessed from anywhere in the script, as they are globally available.
In addition to scalar values, constants in PHP can also be arrays, allowing you to define fixed data structures that remain constant during the execution of the script.
When accessing constants in PHP, you can use direct access or the constant() function, depending on your specific requirements. Direct access is the most common approach and provides a more efficient and concise way to retrieve the constant value. The constant() function is useful when you need to access constants dynamically or when the constant name is stored in a variable.
Overall, constants in PHP are a powerful feature that provides a convenient way to define and manage fixed data values in your PHP code. By using constants, you can avoid hardcoding values in your code and make it more flexible and maintainable.
Check out another blog: