In this blog, we will explore “PHP Tutorial – A Comprehensive Guide to PHP Syntax Overview“. This blog covers various topics, including PHP syntax, writing “Hello, World” in PHP, basic PHP syntax, and PHP tags, etc. Let’s dive in and check it out:
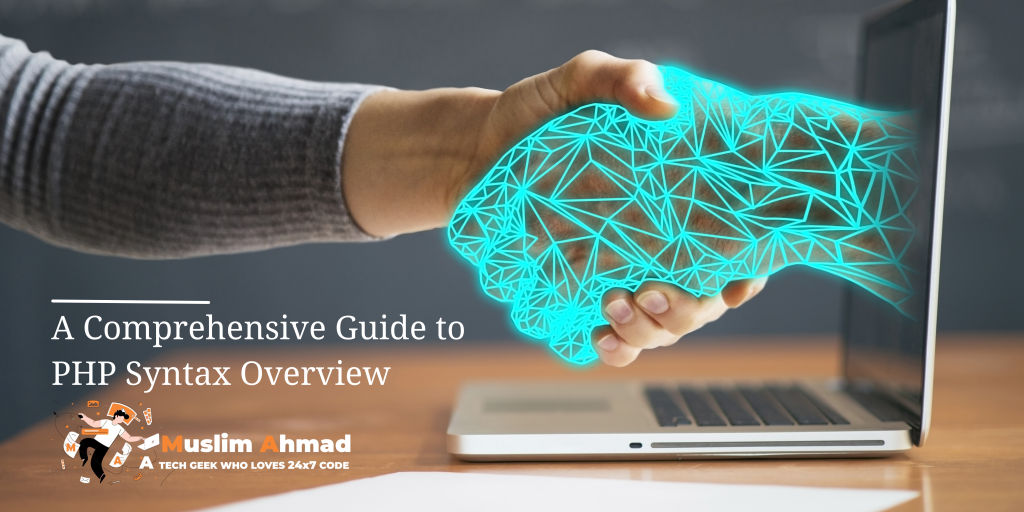
PHP Syntax:
PHP (Hypertext Preprocessor) is a server-side scripting language that is commonly used to create dynamic web pages. It is typically embedded within HTML code, and the PHP code is executed on the server before the HTML is sent to the client’s web browser.
“Hello, World” in PHP Syntax:
The “Hello, World!” program is a classic example that is often used to introduce new programmers to a programming language. In PHP, the “Hello, World!” program is typically written using the echo statement, which outputs a string to the browser. Here is an example of a basic “Hello, World!” program in PHP:
<?php echo "Hello, World!"; ?>
You can also use print instead of echo.
<?php print "Hello, World!"; ?>
When you run this code in a web server that is configured to process PHP code, it will output the string “Hello, World!” to the browser.
You could also include the PHP tags in a HTML page like this,
<!DOCTYPE html> <html> <body> <?php echo "Hello World!"; ?> </body> </html>
You can also use the <?= shorthand for <?php echo to make your code more concise, and this <?= introduce in PHP 5.4.0 version.
<?= "Hello World!" ?>
This will output “Hello World” in the browser.
This basic “Hello, World!” program demonstrates the basics of how to use PHP to output strings to the browser. Of course, in practice, PHP is often used to generate much more complex and dynamic content, but this is a good starting point to understand how to write and run PHP scripts (PHP Syntax).
Basic PHP Syntax:
PHP uses a similar syntax (PHP Syntax) to other programming languages such as C and Java. Here are some basic elements of PHP syntax that you should be familiar with:
- Comments: PHP supports single-line and multi-line comments. A single-line comment is indicated by two forward slashes (//), and a multi-line comment is indicated by a forward slash and an asterisk (/* */). For example:
// This is a single-line comment /* This is a multi-line comment */
- Variables: Variables in PHP are denoted by a dollar sign ($). The basic syntax for declaring a variable is to use the keyword “var” or “let” or simply use the $ sign followed by the name of the variable. It’s recommended to use camelCase for variable names. For example:
$x = 5; $name = "John Doe"; $isStudent = true;
- Data types: PHP syntax supports a wide range of data types, including strings, integers, floating-point numbers, booleans, and arrays. PHP is a loosely typed language which means that you don’t have to explicitly define data types for variables. PHP automatically converts the data type based on the value you assigned to the variable.
$name = "John Doe"; //string $age = 30; //integer $height = 1.8; //float $isStudent = true; //Boolean $class = array("Math", "Science", "English"); // array
- Conditional statements: Conditional statements such as if-else and switch are used to control the flow of the program based on different conditions. For example:
if ($age > 18) { echo "You are an adult"; } else { echo "You are not an adult"; }
switch ($day) { case 1: echo "It's Monday"; break; case 2: echo "It's Tuesday"; break; default: echo "I don't know what day it is"; }
- Loops: PHP syntax supports various loop constructs such as while, do-while, for and foreach. Here’s an example of a while loop:
$i = 0; while ($i < 10) { echo $i; $i++; }
- Functions: PHP has a wide range of built-in functions, and you can also create your own custom functions. Functions are defined using the “function” keyword followed by the name of the function and a set of parentheses. For example:
function greet($name) { echo "Hello, " . $name; } greet("John Doe"); // Outputs: Hello, John Doe
This is a basic overview of the syntax used in PHP. Keep in mind that this is just the tip of the iceberg, and there is much more to learn about the language.
PHP Tags in PHP Syntax:
PHP tags are used to indicate to the server that the enclosed code should be executed as PHP. The default opening tag is <?php, and the closing tag is ?>.
When PHP syntax code is embedded within an HTML or other type of markup file, the PHP code must be enclosed within these tags in order for the server to properly execute the code. For example:
<html> <body> <p>The current date and time is: <?php echo date("Y-m-d H:i:s"); ?></p> </body> </html>
In the example above, the PHP syntax code <?php echo date(“Y-m-d H:i:s”) ?> is used to display the current date and time within a paragraph element.
It’s also worth mentioning that PHP also allows an alternative syntax for opening tags, the short tags <?, <?= and the ASP-style tags <% and %>. However, using these tags is discouraged because they are not always available, depending on the PHP configuration of the server.
It’s recommended to stick to the default tags <?php and ?> for maximum compatibility across different servers and environments.
Keep in mind that when you use <? instead of <?php or <% instead of <%= it may cause issues and errors. This because sometimes <? and <% are used as part of XML or other languages and the server may interpret that as a PHP code, causing errors or unexpected results.
Is PHP Case Sensitive?
In PHP, variable and function names are case-sensitive. This means that a variable named $name is different from a variable named $Name or $NAME. However, keywords such as “if”, “else”, “while”, and “function” are not case-sensitive, so you can write them in uppercase or lowercase and the code will still work.
The following are examples that illustrate the case-sensitivity of variables in PHP:
$name = "John Doe"; $Name = "Jane Doe"; echo $name; // Outputs: John Doe echo $Name; // Outputs: Jane Doe
Additionally, in PHP, constants are case-sensitive by default. When you define a constant using the define() function, the name of the constant must be written in uppercase letters. It’s a common practice in PHP to define constants in all uppercase letters. It’s good practice to be consistent in naming conventions and follow the same case-sensitive format across your code.
define("PI", 3.14); define("GRAVITY", 9.8); echo PI; // Outputs: 3.14 echo gravity; // PHP Notice: Use of undefined constant gravity
Also, be aware that the names of functions and class methods are case-insensitive by default. However, if you are running PHP in a case-sensitive file system, you need to use the same case when calling the function or method as it was defined, otherwise, you will get an “undefined function” error.
function testFunction() { echo "I'm a test function"; } testfunction(); // This will throw an error " Call to undefined function testfunction()" testFunction(); // This will execute the function
By keeping in mind that variables and constants are case-sensitive, whereas keywords and function names are not case-sensitive, you can avoid some common mistakes when writing PHP code.
Conclusion:
In conclusion, PHP is a widely-used server-side scripting language that is commonly used to create dynamic web pages. To use PHP, code must be enclosed within PHP tags, which indicate to the server that the enclosed code should be executed as PHP. The default opening tag is <?php, and the closing tag is ?>.
PHP uses a similar syntax to other programming languages such as C and Java, and supports a wide range of data types, including strings, integers, floating-point numbers, booleans, and arrays.
PHP also supports a wide range of control structures such as conditional statements, loops, and functions. It has many built-in functions and libraries to perform various tasks. You could also use it to write Object Oriented code, create classes, objects and inherit properties and methods.
Keep in mind that variable and constant names are case-sensitive in PHP, and function names are case-insensitive by default.
Overall, PHP is a powerful and versatile language that can be used to create a wide range of web applications, from simple websites to complex web-based systems.
Check out another blog: