In this blog, we will explore “PHP Tutorial – A Comprehensive Guide to PHP Environment Setup”. This tutorial covers all key topics, including Apache setup, Nginx setup, and more. Let’s dive in and check it out:
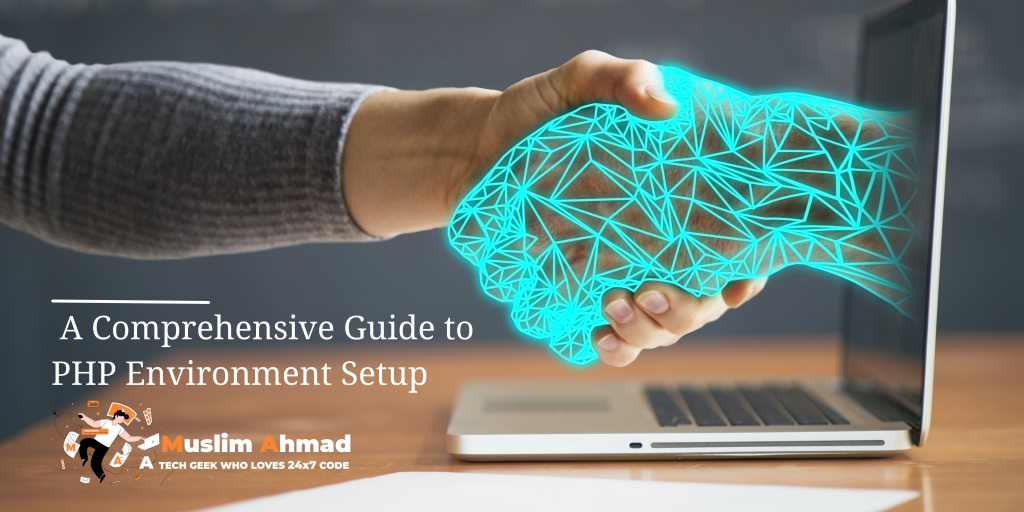
Description – PHP Environment Setup:
To set up a PHP development environment, you will need to install a web server and PHP Environment Setup on your computer. Here’s a general overview of the steps you can follow:
- Install a web server: There are several web servers available, such as Apache and Nginx. Apache is the most widely used web server, and it is usually easy to install on most operating systems.
- Install PHP: Once you have a web server installed, you can install PHP. You can download the latest version of PHP from the PHP website. Follow the instructions provided to install PHP on your system.
- Test your PHP installation: After installing PHP, you can test your installation by creating a PHP file and accessing it through your web server. To do this, create a file called “test.php” and add the following code:
<?php echo "Hello, world!"; ?>
Save the file and place it in the root directory of your web server. Then, open your web browser and navigate to “http://localhost/test.php“. If everything is set up correctly, you should see the message “Hello, world!” displayed in your browser.
- Install a text editor: You will need a text editor to write and edit your PHP code. There are many options available, such as Sublime Text, Atom, and Visual Studio Code. Choose the one that you feel most comfortable with and install it on your system.
- Install any additional libraries or frameworks: Depending on your project requirements, you may need to install additional libraries or frameworks to support your PHP code. For example, if you are using a database, you may need to install a database library like PDO (PHP Data Objects) or MySQLi (MySQL Improved).
Apache Setup – PHP Environment Setup:
To set up Apache with PHP, you will need to install both Apache and PHP on your system. Here’s a general overview of the steps you can follow:
- Download and install Apache: You can download the latest version of Apache from the Apache website. Follow the instructions provided to install Apache on your system.
- Download and install PHP: You can download the latest version of PHP from the PHP website. Make sure to select the version that is compatible with your web server (e.g., Apache 2.4). Follow the instructions provided to install PHP on your system.
- Configure PHP with Apache: After installing PHP, you will need to configure it to work with Apache. To do this, you will need to edit the Apache configuration file (httpd.conf) and add the following lines:
LoadModule php7_module "C:/php/php7apache2_4.dll" AddType application/x-httpd-php .php PHPIniDir "C:/php"
Make sure to replace the paths with the correct paths on your system.
- Restart Apache: After making these changes to the Apache configuration file, you will need to restart Apache for the changes to take effect. You can do this by stopping and starting the Apache service, or by running the “httpd.exe” executable with the “-k restart” flag.
- Test your PHP installation: After installing PHP, you can test your installation by creating a PHP file and accessing it through your web server. To do this, create a file called “test.php” and add the following code:
<?php echo "Hello, world!"; ?>
Save the file and place it in the root directory of your web server. Then, open your web browser and navigate to “http://localhost/test.php“. If everything is set up correctly, you should see the message “Hello, world!” displayed in your browser.
Nginx Setup – PHP Environment Setup:
To set up Nginx to work with PHP on your system, you will need to do the following:
- Download and install Nginx: You can download the latest version of Nginx from the Nginx website. Follow the instructions provided to install Nginx on your system.
- Download and install PHP: You can download the latest version of PHP from the PHP website. Follow the instructions provided to install PHP on your system.
- Configure PHP with Nginx: After installing PHP, you will need to configure it to work with Nginx. To do this, you will need to edit the Nginx configuration file (nginx.conf) and add the following lines:
location ~ \.php$ { include fastcgi_params; fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name; fastcgi_pass 127.0.0.1:9000; }
This will enable Nginx to pass requests for PHP files to the PHP-FPM (FastCGI Process Manager) service, which is responsible for running PHP scripts.
- Configure PHP-FPM: You will also need to configure the PHP-FPM service to listen on a specific port (e.g., 9000) and accept requests from Nginx. You can do this by editing the PHP-FPM configuration file (www.conf) and adding the following lines:
listen = 127.0.0.1:9000 listen.allowed_clients = 127.0.0.1
Make sure to replace the IP address and port number with the correct values for your system.
- Restart Nginx and PHP-FPM: After making these changes to the Nginx and PHP-FPM configuration files, you will need to restart both services for the changes to take effect. You can do this by running the appropriate commands (e.g., “systemctl restart nginx” or “service nginx restart”) or by using the appropriate tools (e.g., the Nginx Control Panel or the PHP-FPM Manager).
- Test your PHP installation: After installing PHP, you can test your installation by creating a PHP file and accessing it through your web server. To do this, create a file called “test.php” and add the following code:
<?php echo "Hello, world!"; ?>
Save the file and place it in the root directory of your web server. Then, open your web browser and navigate to “http://localhost/test.php“. If everything is set up correctly, you should see the message “Hello, world!” displayed in your browser.
Apache2, PHP & MYSQL install in Windows – PHP Environment Setup:
To install Apache2, PHP, and MySQL on a Windows machine, you can follow these steps:
- Download and install Apache: You can download the latest version of Apache from the Apache website. Follow the instructions provided to install Apache on your system.
- Download and install PHP: You can download the latest version of PHP from the PHP website. Make sure to select the version that is compatible with your web server (e.g., Apache 2.4). Follow the instructions provided to install PHP on your system.
- Configure PHP with Apache: After installing PHP, you will need to configure it to work with Apache. To do this, you will need to edit the Apache configuration file (httpd.conf) and add the following lines:
LoadModule php7_module "C:/php/php7apache2_4.dll" AddType application/x-httpd-php .php PHPIniDir "C:/php"
Make sure to replace the paths with the correct paths on your system.
- Download and install MySQL: You can download the latest version of MySQL from the MySQL website. Follow the instructions provided to install MySQL on your system.
- Test your PHP and MySQL installation: After installing PHP and MySQL, you can test your installation by creating a PHP file that connects to a MySQL database and retrieves some data. To do this, create a file called “test.php” and add the following code:
<?php $host = "localhost"; $user = "username"; $password = "password"; $dbname = "database_name"; // Create connection $conn = mysqli_connect($host, $user, $password, $dbname); // Check connection if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } $sql = "SELECT * FROM table_name"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { // output data of each row while($row = mysqli_fetch_assoc($result)) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. "<br>"; } } else { echo "0 results"; } mysqli_close($conn); ?>
Make sure to replace the placeholder values (e.g., “username”, “password”, etc.) with the correct values for your system. Save the file and place it in the root directory of your web server. Then, open your web browser and navigate to “http://localhost/test.php“. If everything is set up correctly, you should see a list of data retrieved from the MySQL database displayed in your browser.
Apache2, PHP & MYSQL install in Linux – PHP Environment Setup:
To install Apache2, PHP, and MySQL on a Linux machine, you can follow these steps:
- Install Apache: Depending on your Linux distribution, you can install Apache using the package manager. For example, on Ubuntu, you can use the following command:
sudo apt-get install apache2
This will install the latest version of Apache and start the Apache service.
- Install PHP: Once you have Apache installed, you can install PHP. Again, depending on your Linux distribution, you can use the package manager to install PHP. For example, on Ubuntu, you can use the following command:
sudo apt-get install php
This will install the latest version of PHP and all the necessary dependencies.
- Install MySQL: To install MySQL, you can again use the package manager. For example, on Ubuntu, you can use the following command:
sudo apt-get install mysql-server
This will install the latest version of MySQL and start the MySQL service.
- Test your Apache, PHP, and MySQL installation: After installing Apache, PHP, and MySQL, you can test your installation by creating a PHP file that connects to a MySQL database and retrieves some data. To do this, create a file called “test.php” and add the code which we have added in windows.
Apache2, PHP & MYSQL install in MAC – PHP Environment Setup:
To install Apache2, PHP, and MySQL on a Mac, you can follow these steps:
- Install Apache: You can install Apache on your Mac using the built-in Apache webserver that comes with macOS. To start the Apache service, open Terminal and enter the following command:
sudo apachectl start
This will start the Apache service and make your website available at “http://localhost“.
- Install PHP: To install PHP on your Mac, you can use the package manager Homebrew. If you don’t have Homebrew installed, you can install it by running the following command in Terminal:
/usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
Once you have Homebrew installed, you can use it to install PHP by running the following command:
brew install php
This will install the latest version of PHP and all the necessary dependencies.
- Install MySQL: To install MySQL, you can again use Homebrew. Run the following command to install MySQL:
brew install mysql
This will install the latest version of MySQL and start the MySQL service.
- Test your Apache, PHP, and MySQL installation: After installing Apache, PHP, and MySQL, you can test your installation by creating a PHP file that connects to a MySQL database and retrieves some data. To do this, create a file called “test.php” and add the code which we have added in windows.
We will discuss XAMPP, WAMP, LAMP, MAMP & IIS Server Setup for PHP Environment in more detail in the next chapters.
Check out another blog: